既存のプロジェクトにJetpack Composeを導入してみた所、公式資料通りではビルドが通りませんでした…
試行錯誤で動くように成ったので、その手順を解説します。
今後の環境設定の参考になるよう行ったり来たりの手順も記載していますので、結果だけ知りたい人は最後の節「4.まとめ」を見てみてください。
これ通りやっても動かないですが、参考として公式リンクも貼っておきます。
https://developer.android.com/jetpack/compose/setup
1. AndroidStudioを最新に
2023/10/28時点で最新はAndroidStudio Giraffe 2022.3.1 Patch2でした。
入れるといつも通りgradleやら何やらアップデートを促されるので最新に更新します。
gradle8.1.2になりました。
2. Jetpack Composeを有効にする
Moduleレベルのbuild.gradleに次の設定を追記します。
android {
buildFeatures {
compose true
}
composeOptions {
kotlinCompilerExtensionVersion = "1.5.3"
}
}
ここで一旦ビルドしてみると次のエラー
e: This version (1.3.2) of the Compose Compiler requires Kotlin version 1.7.20 but you appear to be using Kotlin version 1.6.21 which is not known to be compatible. Please fix your configuration (or suppressKotlinVersionCompatibilityCheck but don’t say I didn’t warn you!).
(最新のAndroidStudioに最初から入ってたJetpack Compose 1.3.2は、Kotlin 1.7.20が必要だけど、あんたのは1.6.21だぞ。
アップデートしろ!)
エラーの指示通り、kotlinをアップデートするため、Projectレベルのbuild.gradleにあるkotlinプラグインのバージョンを変更します。
- classpath 'org.jetbrains.kotlin:kotlin-gradle-plugin:1.6.21'
+ classpath 'org.jetbrains.kotlin:kotlin-gradle-plugin:1.7.20'
もう一度ビルドしてみると次のエラー
androidx.compose.compiler.plugins.kotlin.IncompatibleComposeRuntimeVersionException: The Compose Compiler requires the Compose Runtime to be on the class path, but none could be found. The compose compiler plugin you are using (version 1.3.2) expects a minimum runtime version of 1.0.0. at androidx.compose.compiler.plugins.kotlin.VersionChecker.noRuntimeOnClasspathError(VersionChecker.kt:151)
(最新のAndroidStudioに最初から入ってたJetpack Compose 1.3.2は、Compose Compilerが必要だけど無いわ。)
無いなら入れましょう。
(参考:https://www.appsloveworld.com/kotlin/100/6/the-compose-compiler-plugin-you-are-using-version-1-0-0-alpha13-expects-a-minim?expand_article=1)
Moduleレベルのbuild.gradleに追加します。最新の1.5.4をば。
dependencies {
implementation("androidx.compose.runtime:runtime:1.5.4")
}
改めてビルドすると次のエラー
java.lang.Error: Something went wrong while checking for version compatibility between the Compose Compiler and the Kotlin Compiler. It is possible that the versions are incompatible. Please verify your kotlin version and consult the Compose-Kotlin compatibility map located at https://developer.android.com/jetpack/androidx/releases/compose-kotlin
(Kotlinのバージョンが、AndroidStudioに入ってるJetpack Composeのバージョンに対応してないっぽいわ。リンクにある、ComposeとKotlinの対応バージョン一覧を確認してみて)
リンクを見てみると… (https://developer.android.com/jetpack/androidx/releases/compose-kotlin)
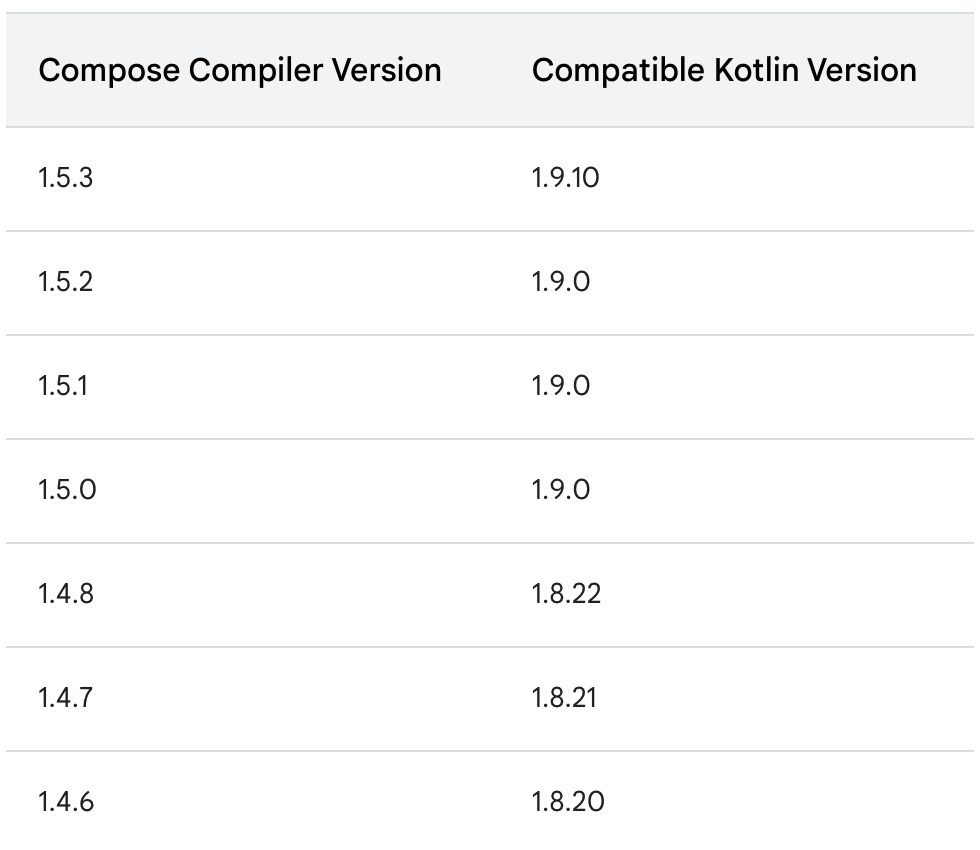
さっき入れたcompose compilerのバージョンを1.5.4にしたけど(“androidx.compose.runtime:runtime:1.5.4”)、1.5.3までしか表にないので、1.5.3に落としつつ、さらにもっと前にkotlin:kotlin-gradle-pluginのバージョンを1.7.2に上げたけど、指定リンク先の表通り、1.9.10に上げます。
Moduleレベルのbuild.gradle
- implementation("androidx.compose.runtime:runtime:1.5.4")
+ implementation("androidx.compose.runtime:runtime:1.5.3")
Projectレベルのbuild.gradle
classpath "org.jetbrains.kotlin:kotlin-gradle-plugin:1.9.10"
もう一度ビルドすると、無事成功します!
3. 必要なプラグインを追加する
後は用途に合わせて必要なプラグインをModuleレベルのbuild.gradleに追加します。
dependencies {
// これ入れないとエラー
implementation("androidx.compose.runtime:runtime:1.5.3")
// Composeの基本となる共通ライブラリーが入っているので入れないとmaterial3とかも使えなくなる
def composeBom = platform('androidx.compose:compose-bom:2023.10.01')
implementation composeBom
androidTestImplementation composeBom
// TextとかUIパーツ系
implementation 'androidx.compose.material3:material3'
// IDE上でプレビューする機能
debugImplementation 'androidx.compose.ui:ui-tooling'
implementation 'androidx.compose.ui:ui-tooling-preview'
// これ入れないとsetContent使えない
implementation 'androidx.activity:activity-compose:1.7.0'
}
この後、空のActivityを作成して、親クラスをComponentActivityに変更し、setContent内にComposeのコードを書くと、無事Jetpack Composeデビュー完了です!
class PassCodeActivity : ComponentActivity() { // ComponentActivityに変更
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
// このカッコ内にComposeのコードを書く
setContent {
Text("パスコード画面ですよ")
}
}
}
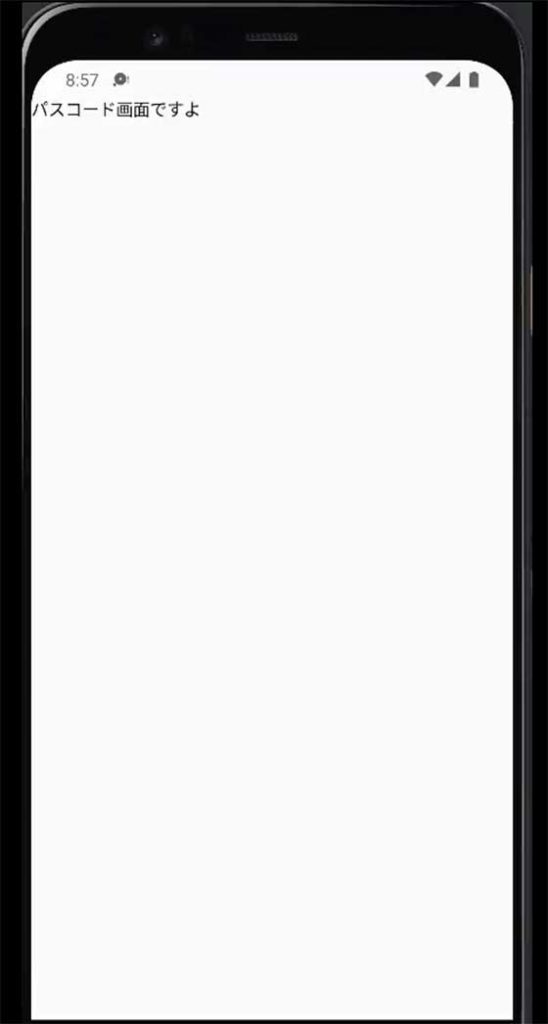
4. まとめ
試行錯誤が入ったので、最後に結局どうすれば動くのかの結論をまとめておきます。
- AndroidStudioを最新にする
(ここでは、AndroidStudio Giraffe 2022.3.1 Patch2) - gradleのバージョンを最新にする
(ここでは、8.1.2) - Moduleレベルのbuild.gradleに次を追加してComposeをONにする
android { buildFeatures { compose true } composeOptions { kotlinCompilerExtensionVersion = "1.5.3" } }
- Projectレベルのbuild.gradleに書いているKotlinプラグインのバージョンを1.9.10にする
classpath "org.jetbrains.kotlin:kotlin-gradle-plugin:1.9.10"
- 用途に応じて、Moduleレベルのbuild.gradleにプラグインを追加する。
dependencies { // これ入れないとエラー implementation("androidx.compose.runtime:runtime:1.5.3") // Composeの基本となる共通ライブラリーが入っているので入れないとmaterial3とかも使えなくなる def composeBom = platform('androidx.compose:compose-bom:2023.10.01') implementation composeBom androidTestImplementation composeBom // TextとかUIパーツ系 implementation 'androidx.compose.material3:material3' // IDE上でプレビューする機能 debugImplementation 'androidx.compose.ui:ui-tooling' implementation 'androidx.compose.ui:ui-tooling-preview' // これ入れないとsetContent使えない implementation 'androidx.activity:activity-compose:1.7.0' }